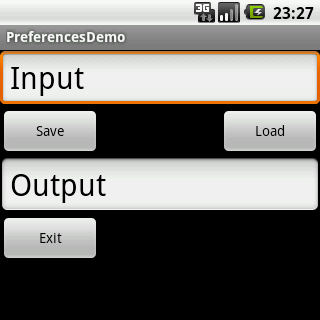
Preference Demo
在Android上儲存資料的方式之一,是使用Preference來儲存,雖然這個類別看起來好像只能儲存"偏好設定",但其實可以依你的需求來儲存少量化的任何資料。SharedPreferences pref = getSharedPreferences("自訂名稱", 0);
接著你就能存入資料:
pref.edit().putString("資料關鍵字key", "資料內容value").commit();
除了可以 put 字串外,還有數字及布林值可以儲存。接著就可以取出資料:
String info = pref.getString("資料關鍵字key", "在取不到資料時的預設值");
就這麼簡單。記得 put 字串就要用相對應的 get 字串方法來取資料,例如 putInt 就要用 getInt 來取。
範例解說
如截圖所示,我建立了 2 個文字輸入區,3 個按鈕。Save 按鈕會把上方的文字輸入區中的文字存入 Preference,Load 按鈕會把 Preference 中的資料取出並在下方的文字輸入區顯示。當按下 Exit 按鈕把 App 關閉後,當 App 再度開啟時,直接按下 Load 按鈕,資料就會直接取回並在下方文字區顯示。範例程式碼
package com.tonycube.demo;
import android.app.Activity;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.TextView;
public class PreferencesDemoActivity extends Activity implements OnClickListener {
private final String PREF_SETTING = "SETTING";
private SharedPreferences pref = null;
private TextView txtInput = null;
private TextView txtOutput = null;
private Button btnSave = null;
private Button btnLoad = null;
private Button btnExit = null;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
initView();
}
private void initView(){
txtInput = (TextView) findViewById(R.id.txtInput);
txtOutput = (TextView) findViewById(R.id.txtOutput);
btnSave = (Button) findViewById(R.id.btnSave);
btnLoad = (Button) findViewById(R.id.btnLoad);
btnExit = (Button) findViewById(R.id.btnExit);
btnSave.setOnClickListener(this);
btnLoad.setOnClickListener(this);
btnExit.setOnClickListener(this);
pref = getSharedPreferences(PREF_SETTING, 0);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.btnSave:
pref.edit().putString("info", txtInput.getText().toString()).commit();
break;
case R.id.btnLoad:
String info = pref.getString("info", "default value");
txtOutput.setText(info);
break;
case R.id.btnExit:
finish();
break;
default:
break;
}
}
}
請教一下,如果想用editText 輸入的文字去建一個Preference
回覆刪除不知道能不能達成
可以,做法和範例一樣
刪除