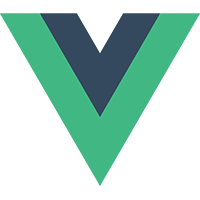
Vue.js
Laravel 允許我們在 Artisan 中加入自己的指令,而我們每次在建立一個 Vue 元件檔時,動作及內容都差不多,這些重覆的流程就應該把它們自動化。建立指令
首先,我們來看看 Artisan 提供了哪些指令,執行:php artisan list
在 make
那一項,我們將會建立一個 make:vue
這樣的指令來幫我們產生 Vue 元件檔。其中你應該有注意到,Artisan 有提供「產生指令」的指令,執行它:
php artisan make:command MakeVueComponent
會產生 app/Console/Commands/MakeVueComponent.php
檔,打開它會看到幾個屬性及方法:
signature
指令的簽名,也就是你的指令要怎麼用,請將它改成:protected $signature = 'make:vue {name} {--nojs : Do not create a js file}';
{name}
將會在接受參數後產生該名稱的 Vue 元件檔。{--nojs}
是額外參數,可以指定不要產生相對應的 js 檔。冒號後面是該參數的說明,可省略。
description
指令的說明,當你使用php artisan list
時會看到的說明,請將它改成:
protected $description = 'Create a new Vue Component';
handle()
當指令被執行時呼叫的方法。完整的 MakeVueComponent.php 內容
php<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
use Illuminate\Filesystem\Filesystem;
/**
* @author: Tony Blog
* @site: http://blog.tonycube.com
*/
class MakeVueComponent extends Command
{
/**
* The file path of the vue component.
*/
const VUE_COMPONENT_PATH = 'resources/assets/js/components/';
/**
* The file path of the vue js.
*/
const VUE_JS_PATH = 'resources/assets/js/';
/**
* The stub of the vue component.
*/
const VUE_COMPONENT_STUB = __DIR__.'/stubs/vue.stub';
/**
* The stub of the vue js.
*/
const VUE_JS_STUB = __DIR__.'/stubs/vuejs.stub';
/**
* The name and signature of the console command.
*
* @var string
*/
protected $signature = 'make:vue {name} {--nojs : Do not create a js file}';
/**
* The console command description.
*
* @var string
*/
protected $description = 'Create a new Vue Component';
/**
* The object of the Filesystem.
*
* @var Filesystem
*/
protected $fs;
/**
* Create a new command instance.
*
* @return void
*/
public function __construct(Filesystem $fs)
{
parent::__construct();
$this->fs = $fs;
}
/**
* Execute the console command.
*
* @return mixed
*/
public function handle()
{
$this->createVueComponent();
$this->createVueJS();
}
private function createVueComponent()
{
$name = $this->argument('name');
$path = base_path(self::VUE_COMPONENT_PATH . $name).'.vue';
if ($this->fs->exists($path)) {
$this->error('Vue component already exists!');
return;
}
//
$this->makeDirectory($path);
$stub = $this->fs->get(self::VUE_COMPONENT_STUB);
$this->fs->put($path, $stub);
$this->info('Vue component created successfully.');
}
private function createVueJS()
{
$nojs = $this->option('nojs');
if (! $nojs) {
$name = $this->argument('name');
$path = base_path(self::VUE_JS_PATH . strtolower($name)).'.js';
if ($this->fs->exists($path)) {
$this->error('Vue js already exists!');
return;
}
//
$this->makeDirectory($path);
$stub = $this->fs->get(self::VUE_JS_STUB);
$stub = $this->renderStub($stub, ['name' => $name]);
$this->fs->put($path, $stub);
$this->info('Vue js created successfully.');
}
}
private function makeDirectory(string $path)
{
if (! $this->fs->isDirectory(dirname($path))) {
$this->fs->makeDirectory(dirname($path), 0777, true, true);
}
}
private function renderStub($stub, $datas)
{
foreach ($datas as $find => $replace) {
$stub = str_replace('$'.$find, $replace, $stub);
}
return $stub;
}
}
說明:
- 2 個 PATH 常數是指定輸出檔案時的目錄。
- 2 個 STUB 常數是指定範本檔的所在路徑。
- 因為要輸出檔案,所以必須載入 FileSystme 類別。
- handle() 中,我們只做 2 件事,輸出 Vue component 檔及相對應的 js 檔。
- renderStub() 是用來置換掉範本中指定位置的(佔位)內容。
2. 建立範本 (Stub)
建立app/Console/Commands/stubs/vue.stub
:
Vue<template>
<div class="content">
</div>
</template>
<script>
export default {
data() {
return {
}
}
}
</script>
<style>
</style>
建立 app/Console/Commands/stubs/vuejs.stub
:
JavaScriptrequire('./bootstrap');
import $name from './components/$name'
new Vue({
el: '#app',
components: { $name }
})
這裡的 $name
會被前面所說的 renderStub() 給置換成指定的內容。
3. 註冊指令
編輯app/Console/Commands/Kernel.php
:
PHPprotected $commands = [
Commands\MakeVueComponent::class
];
將我們的指令加入,這樣就完成了。
4. 使用指令
現在,可以使用我們的指令來產生 Vue 元件檔了,執行:php artisan make:vue Home
會產 resources/assets/js/commponents/Home.vue
及 resources/assets/js/home.js
。如果你不想產生
home.js
,可以加上 --nojs
即可。
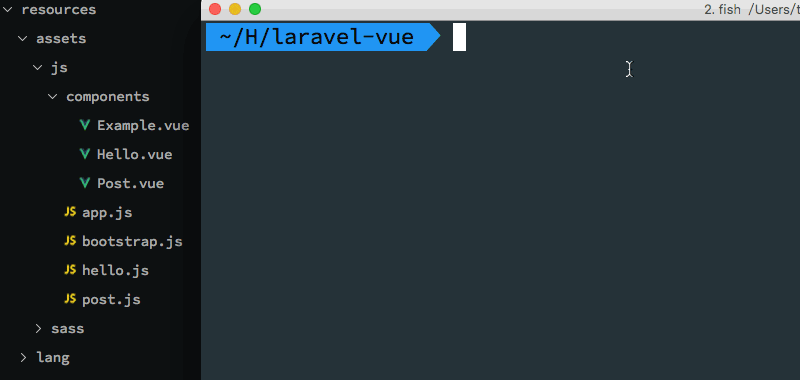
參考資料
本文網址:https://blog.tonycube.com/2017/06/vuejs-13-laravel-artisan-vue.html
由 Tony Blog 撰寫,請勿全文複製,轉載時請註明出處及連結,謝謝 😀
由 Tony Blog 撰寫,請勿全文複製,轉載時請註明出處及連結,謝謝 😀
請教一下,該如何將上述動作包成一個 package 供人做利用(composer require Vue/vue)。
回覆刪除敝人是使用 Laravel 做開發。
你可以研究一下 composer 的套件
刪除https://getcomposer.org/doc/02-libraries.md